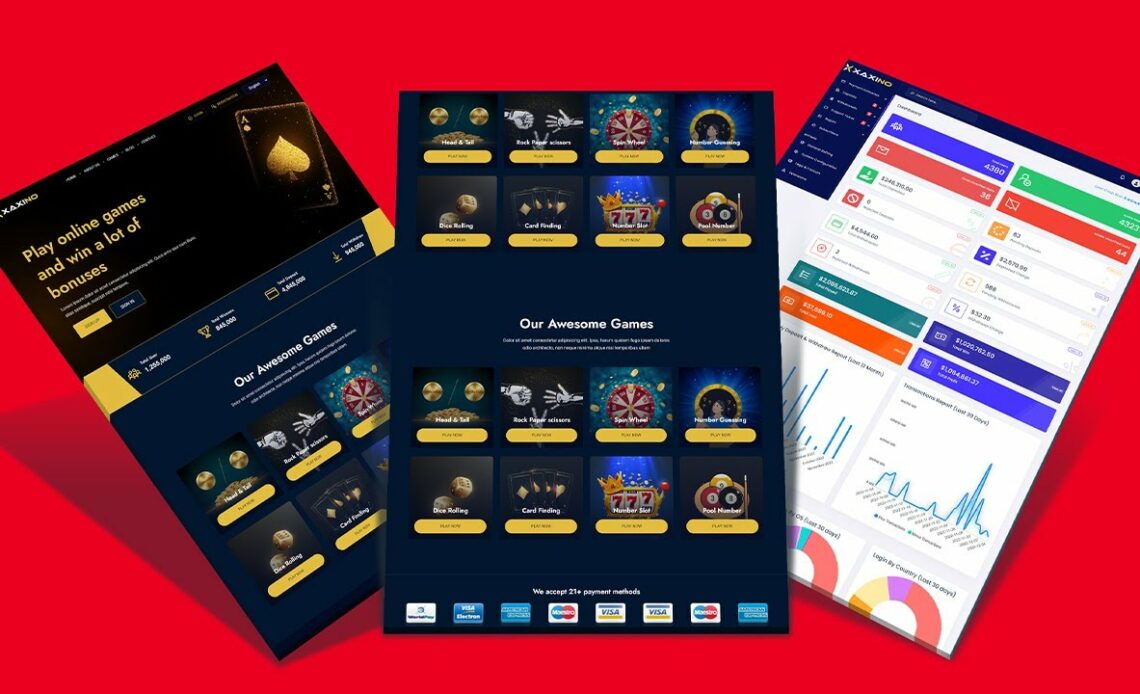
PHP, or Hypertext Preprocessor, is a widely-used server-side scripting language, especially for developing web-based applications. In the context of online casinos and slot games (like this popular slot-game), PHP plays a critical role in delivering interactive, scalable, and secure gaming platforms.
While modern frameworks and technologies such as JavaScript, Node.js, or Python have gained popularity in some areas, PHP remains a dominant player in the casino industry for several reasons.
This article will explore the features of PHP that make it an ideal choice for online casino platforms, focusing on slot game development, backend functionality, database interactions, security considerations, and examples of common use cases in this industry.
1. Why PHP is Preferred in Online Casinos
PHP has been a cornerstone for web development due to its simplicity, flexibility, and ability to scale web applications. Here are several key reasons why PHP is a top choice for online casino platforms and slot game development:
a. Seamless Server-Side Execution
Online casinos rely on server-side scripting to handle the complex operations behind the scenes. PHP is well-suited for such tasks because it executes on the server and returns the final HTML to the client. This means that sensitive information, such as payment processing and player data, is securely managed on the server, reducing the risk of exposure.
For example, when a player interacts with a slot game or places a bet, PHP scripts handle the request, interact with the database to retrieve or update data (e.g., balance, win/loss status), and then render the result on the player’s screen.
b. Integration with Databases
Online casinos typically handle vast amounts of data, from user accounts to transaction logs and game statistics. PHP integrates smoothly with databases such as MySQL, PostgreSQL, and MariaDB, making it easier to manage this data.
Here’s a simple example of how PHP handles database interaction for user account verification:
<?php
// Connection to the casino's database
$host = "localhost";
$dbname = "casino";
$user = "root";
$password = "password";
$conn = new PDO("mysql:host=$host;dbname=$dbname", $user, $password);
// Fetch player details
$sql = "SELECT * FROM players WHERE player_id = :player_id";
$stmt = $conn->prepare($sql);
$stmt->bindParam(':player_id', $_GET['player_id']);
$stmt->execute();
$player = $stmt->fetch();
if ($player) {
echo "Welcome, " . $player['username'];
} else {
echo "Player not found.";
}
?>
This simple example shows how PHP connects to a database, retrieves user information, and displays it securely on the page. It is crucial for verifying login credentials, managing player profiles, and tracking game performance.
c. Session Management
PHP’s robust session handling makes it ideal for managing users’ activity on online casinos. It allows the platform to keep track of a player’s login status, game progress, and betting history. Since online casinos handle multiple simultaneous sessions, PHP’s built-in session management features allow seamless transitions between different games and activities.
For example, when a user logs into an online casino, PHP uses sessions to track the user’s balance, any free spins or bonuses, and even save their progress on certain slot games. This ensures a personalized experience for each player across multiple games.
<?php
session_start();
$_SESSION['username'] = 'john_doe';
$_SESSION['balance'] = 100.00;
// Accessing session variables during gameplay
echo "User: " . $_SESSION['username'] . " has a balance of $" . $_SESSION['balance'];
?>
This snippet shows how PHP sessions help store and retrieve data between different page requests, crucial for online casino applications.
2. Real-Time Operations for Slot Games
a. Random Number Generation (RNG)
One of the most critical elements in slot game development is the Random Number Generator (RNG), which ensures fair play by generating random outcomes for every spin. PHP can interface with external RNG libraries or use internal functions like mt_rand()
to produce random results.
Here’s an example of a basic RNG logic for a slot machine developed using PHP:
<?php
// Define slot machine symbols
$symbols = ["Cherry", "Bell", "Bar", "Seven", "Diamond"];
// Simulate a spin
$reel1 = $symbols[mt_rand(0, count($symbols) - 1)];
$reel2 = $symbols[mt_rand(0, count($symbols) - 1)];
$reel3 = $symbols[mt_rand(0, count($symbols) - 1)];
// Display results
echo "You got: $reel1 | $reel2 | $reel3";
?>
This basic script simulates a simple slot machine with three reels, where the outcomes are randomly selected. However, in a real-world online casino, the RNG mechanism is far more complex and secure, usually integrating certified RNG libraries to comply with industry standards.
b. Asynchronous Features for Real-Time Updates
PHP can work with asynchronous technologies such as WebSockets or integrate with AJAX to provide real-time updates. For example, in online casinos, it’s essential to display real-time winnings, jackpot updates, and leaderboards. Although PHP is primarily synchronous, it can be integrated with JavaScript or Node.js to provide asynchronous capabilities.
// Example: Using AJAX for real-time jackpot updates
$.ajax({
url: 'get_jackpot.php',
success: function(data) {
$('#jackpot_amount').html(data);
}
});
This allows online casinos to keep players engaged with live information without having to refresh the page.
3. Security Considerations in PHP for Online Casinos
Online casinos handle sensitive data, including financial transactions and personal information. Security is paramount, and PHP offers several built-in features that help developers secure their applications.
a. Input Validation and Sanitization
To prevent SQL Injection and XSS (Cross-Site Scripting) attacks, PHP provides functions such as filter_var()
, htmlspecialchars()
, and prepared statements. These tools are crucial for sanitizing user input and ensuring that malicious data does not compromise the system.
// Example: Prepared Statement to prevent SQL Injection
$stmt = $conn->prepare("SELECT * FROM players WHERE username = :username");
$stmt->bindParam(':username', htmlspecialchars($_POST['username']));
$stmt->execute();
This protects the database from unauthorized access and manipulation by ensuring that the data is properly sanitized before executing SQL queries.
b. HTTPS and Encryption
PHP works seamlessly with SSL certificates to encrypt data transmission between the server and the client. This ensures that sensitive information, such as credit card details, remains secure during online transactions.
In addition to HTTPS, PHP offers OpenSSL functions to implement encryption for sensitive data stored on the server. This is vital in the online casino industry, where payment data must be securely handled to comply with regulations such as PCI DSS (Payment Card Industry Data Security Standard).
// Example: Encrypting sensitive data using OpenSSL in PHP
$encrypted_data = openssl_encrypt($data, 'AES-256-CBC', $encryption_key, 0, $iv);
4. Compliance with Gambling Regulations
Compliance is a major aspect of operating an online casino, and PHP facilitates this by integrating with third-party APIs for KYC (Know Your Customer), AML (Anti-Money Laundering), and fraud prevention. By interfacing with regulatory APIs, PHP applications can automatically verify user identities, track suspicious activities, and generate reports for regulatory bodies.